According to the latest top 25 Software Errors from SANS Institute, overflow integers is one of the security issues we coders need to handle better. At the wrong occasion it can be used to make loops infinitive or not run at all. Also it can be used to make an addition result in a subtraction and vice versa. Making this error available for malicious intent and a security concern for us developers.
Everything in a computer is stored as ones and zeros and so also integers number. When storing an integer in the memory it starts on all the bits set to zero for positive numbers (starting at 0) and all bits set to one for negative numbers (starting at -1), then they are counted up/down and meets in the middle, that is where the overflow can happen. Let’s illustrate this with an example with a low interval. A 2 bit integer, that would range from -2 to 1. Bit 00 would be o and bit 11 would be -1, then 01 would be 1 and 10 would be -2. Now all the bit combinations are taken.
00 = 0 | 01 = 1 | 10 = -2 | 11 = -1
So when adding one to 01 (1) we will go to 10 and that is equal to -2 not 2. 2 isn’t even in the range (-2 to 1) for this very small integer. The same thing for removing one from 10 (-2), that will not be -3 as it’s not in range for the integer but it will be 1.
The keyword int or the structure Integer (they are the same) that you mostly use is a 32 bit integer and can hold integers values in the range from -2 147 483 648 to 2 147 483 647 but the principle is the same as for the imagine 2 bit integer. Note that the unsigned integer, that only have positive numbers still have this “feature” but it will overflow to 0 instead of the most negative number.
Other kinds of number types
Numbers that is stored as floating numbers (like double) will not suffer from this since they are stored in an other way. They will only suffer from losing precision instead. The datatype decimal on the other hand stores floating number in a precisely way but will not overflow it’s values, instead it will throw an OverflowException. If you calculate your integer with an double or decimal it will be converted to that type and behave the same, but when doing calculation with only integers you need to be careful especially if one of the numbers originates from an external resource.
The solution is the keyword: checked
What you can do is to add the keyword checked to your code to make sure that if a overflow occur there will be a OverlowException thrown instead.
var r = checked(i + 2);
checked
{
var r = i + 2;
}
example before checked:
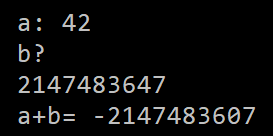
example after checked been added:

Last option - compiler
One other option is the state for the compiler that always make checks for overflows. But the mechanism of overflow checking is heavy on the performance and isn’t really recommended. You can do this by a complier flag or in the properties for your project. And if you do this you can use the keyword unchecked to force the runtime to skip the overflow checking.
This blogpost is a part of The Third Annual C# Advent